API reference
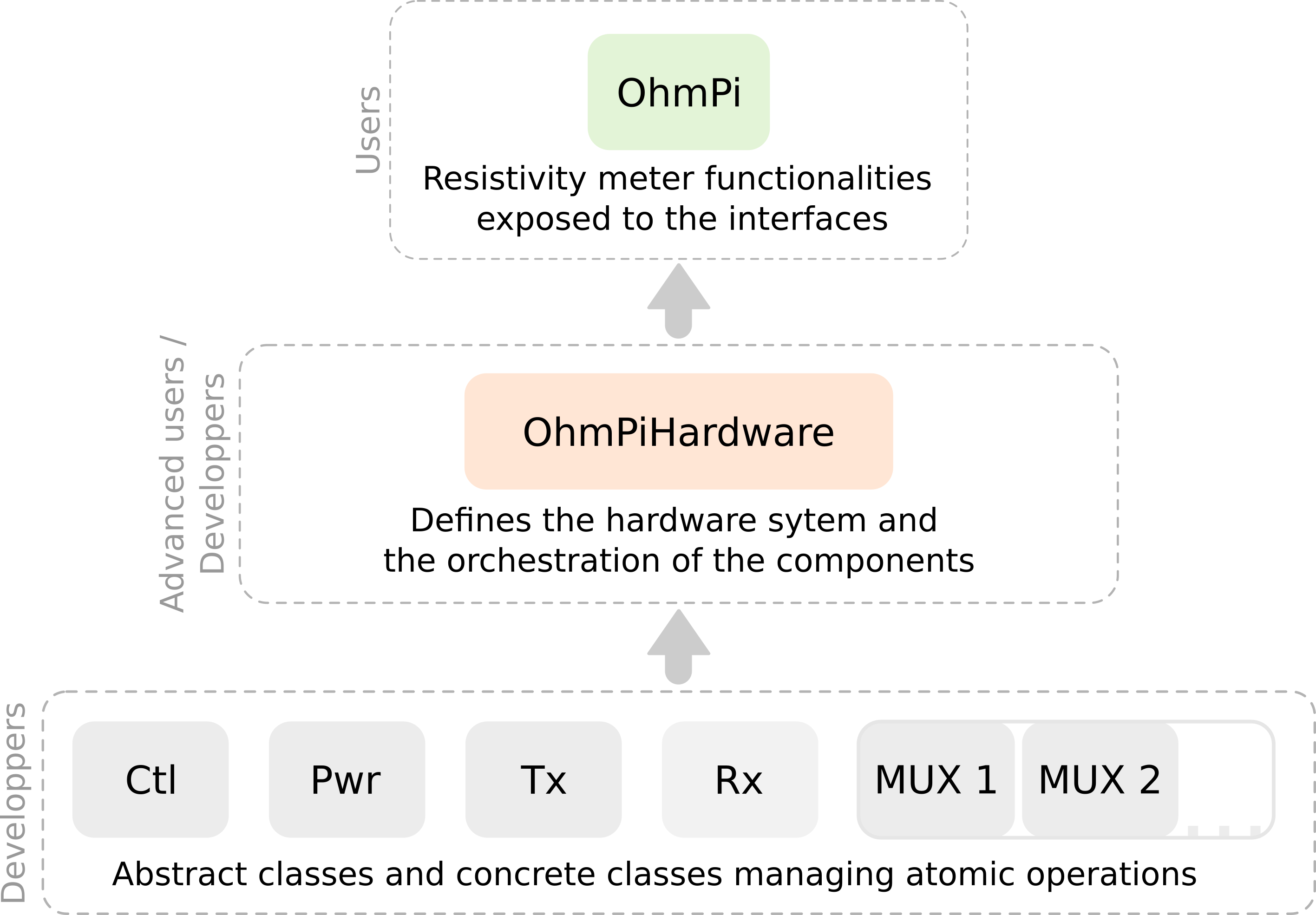
created on January 6, 2020. Updates dec 2023; in-depth refactoring May 2023. Hardware: Licensed under CERN-OHL-S v2 or any later version Software: Licensed under the GNU General Public License v3.0 Ohmpi.py is a program to control a low-cost and open hardware resistivity meters within the OhmPi project by Rémi CLEMENT (INRAE), Vivien DUBOIS (INRAE), Hélène GUYARD (IGE), Nicolas FORQUET (INRAE), Yannick FARGIER (IFSTTAR) Olivier KAUFMANN (UMONS), Arnaud WATLET (UMONS) and Guillaume BLANCHY (FNRS/ULiege).
- class ohmpi.ohmpi.OhmPi(settings=None, sequence=None, mqtt=True, config=None)
OhmPi class.
- Attributes:
sequence
Gets sequence
Methods
append_and_save
(filename, last_measurement)Appends and saves the last measurement dict.
create_sequence
(nelec[, params, ...])Creates a sequence of quadrupole.Several type of sequence or sequence with different parameters can be combined together.
download_data
([start_date, end_date, ftype, ...])Create a zip of the data folder to then download it easily.
export
([fnames, outputdir, ftype, ...])Export surveys stored in the 'data/' folder into an output folder.
find_optimal_vab_for_sequence
([which, n_samples])Find optimal Vab based on sample sequence in order to run sequence with fixed Vab.
get_data
([survey_names, full, cmd_id])Get available data.
interrupt
([cmd_id])Interrupts the acquisition.
load_sequence
(filename[, cmd_id])Reads quadrupole sequence from file.
plot_last_fw
([save_fig, filename])Plots last full waveform measurement
quit
([cmd_id])Quits OhmPi.
remove_data
([cmd_id])Remove all data in the ´export_path´ folder on the raspberrypi.
repeat_sequence
(**kwargs)Identical to run_multiple_sequences().
reset_mux
([cmd_id])Switches off all multiplexer relays.
restart
([cmd_id])Restarts the Raspberry Pi.
rs_check
([vab, cmd_id, couple, tx_volt])Checks contact resistances.
run_inversion
([survey_names, elec_spacing])Run a simple 2D inversion using ResIPy (https://gitlab.com/hkex/resipy).
run_measurement
([quad, nb_stack, ...])Measures on a quadrupole and returns a dictionary with the transfer resistance.
run_multiple_sequences
([sequence_delay, ...])Runs multiple sequences in a separate thread for monitoring mode.
run_sequence
([fw_in_zip, cmd_id, ...])Runs sequence synchronously (=blocking on main thread).
run_sequence_async
([cmd_id])Runs the sequence in a separate thread. Can be stopped by 'OhmPi.interrupt()'.
set_sequence
([sequence, cmd_id])Sets the sequence to acquire.
set_time
(date[, cmd_id])Set date of the RPI remotely.
shutdown
([cmd_id])Shutdown the Raspberry Pi.
switch_mux_off
(quadrupole[, cmd_id])Switches off multiplexer relays for given quadrupole.
switch_mux_on
(quadrupole[, bypass_check, cmd_id])Switches on multiplexer relays for given quadrupole.
test
([test_names, remote, filename])Run test on the ohmpi system.
test_mux
([activation_time, mux_id, cmd_id])Interactive method to test the multiplexer boards.
update_settings
(settings[, cmd_id])Updates acquisition settings from a json file or dictionary.
- append_and_save(filename: str, last_measurement: dict, fw_in_zip=None, cmd_id=None)
Appends and saves the last measurement dict.
- Parameters:
- filenamestr
Filename of the .csv.
- last_measurementdict
Last measurement taken in the form of a python dictionary.
- fw_in_zipbool, optional
Wether to save the full-waveform data in a separate .csv in long format to be zipped to spare space. If None, default is read from default.json.
- cmd_idstr, optional
Unique command identifier.
- create_sequence(nelec, params=[('dpdp', 1, 8)], include_reciprocal=False, opt_ip=False, opt_param={}, opt_plot=False, fpath=None)
Creates a sequence of quadrupole.Several type of sequence or sequence with different parameters can be combined together.
- Parameters:
- nelecint
Number of electrodes.
- paramslist of tuple, optional
Each tuple is the form (<array_name>, param1, param2, …) Dipole spacing is specified in terms of “number of electrode spacing”. Dipole spacing is often referred to ‘a’. Number of levels is a multiplier of ‘a’, often referred to ‘n’. For multigradient array, an additional parameter ‘s’ is needed. Types of sequences available are : - (‘wenner’, a) - (‘dpdp’, a, n) - (‘schlum’, a, n) - (‘multigrad’, a, n, s) By default, if an integer is provided for a, n and s, the parameter will be considered varying from 1 to this value. For instance, for (‘wenner’, 3), the sequence will be generated for a = 1, a = 2 and a = 3. If only some levels are desired, the user can use a list instead of an int. For instance (‘wenner’, [3]) will only generate quadrupole for a = 3.
- include_reciprocalbool, optional
If True, will add reciprocal quadrupoles (so MNAB) to the sequence.
- opt_ipbool, optional
If True, will optimize for induced polarization measurement (i.e. will try to put as much time possible between injection and measurement at the same electrode). Optimization can take a few seconds.
- opt_paramdic, optional
Dictionary of parameters to be passed to optimize_ip(). Possible values are ‘niter’ (int): number of iterations during optimization ‘nchains’ (int): number of chain to run in parallel (each chain is run niter times) ‘pad’ (int): how far from its position move the quad with the largest cost in the sequence
- opt_plotbool, optional
Plot cost decay of ip optimization.
- fpathstr, optional
Path where to save the sequence (including filename and extension). By default, sequence is saved in ohmpi/sequences/sequence.txt.
- download_data(start_date=None, end_date=None, ftype='ohmpi', elec_spacing=1, cmd_id=None)
Create a zip of the data folder to then download it easily.
- Parameters:
- start_datestr, optional
Start date as ISO string (e.g. “2024-12-24”).
- end_datestr, optional
End date as ISO string.
- ftypestr, optional
Format type. Default is OhmPi normal format. Can choose between: - ohmpi (default) - bert (same as pygimli) - pygimli (same as bert) - protocol (for resipy/r2 codes)
- elec_spacingfloat, optional
For some format (e.g. bert, pygimli), electrode position is required.
- export(fnames=None, outputdir=None, ftype='bert', elec_spacing=1, fname_coord=None)
Export surveys stored in the ‘data/’ folder into an output folder.
- Parameters:
- fnameslist of str, optional
List of path (not filename) to survey in ohmpi format to be converted.
- outputdirstr, optional
Path of the output directory where the new files are stored. If None, a directory called ‘output’ is created in OhmPi.
- ftypestr, optional
Type of export. To be chosen between: - bert (same as pygimli) - pygimli (same as bert) - protocol (for resipy, R2 codes)
- elec_spacingfloat, optional
Electrode spacing in meters. Same electrode spacing is assumed.
- find_optimal_vab_for_sequence(which='mean', n_samples=10, **kwargs)
Find optimal Vab based on sample sequence in order to run sequence with fixed Vab. Returns Vab.
- Parameters:
- whichstr
Which vab to keep, either “min”, “max”, “mean” (or other similar numpy method e.g. median) If applying strategy “fixed” based on vab_opt, safer to chose “min”
- n_samples: int
Number of samples to keep within loaded sequence.
- kwargsdict, optional
kwargs passed to Ohmpi.run_sequence.
- Returns:
- Vab_optfloat [in V]
Optimal Vab value
- get_data(survey_names=None, full=False, cmd_id=None)
Get available data.
- Parameters:
- survey_nameslist of str, optional
List of filenames already available from the html interface. So their content won’t be returned again. Only files not in the list will be read.
- fullbool, optional
If False, will only return the quadrupole and transfer resistance (default). If True, will return all columns.
- cmd_idstr, optional
Unique command identifier.
- interrupt(cmd_id=None)
Interrupts the acquisition.
- Parameters:
- cmd_idstr, optional
Unique command identifier.
- load_sequence(filename: str, cmd_id=None)
Reads quadrupole sequence from file.
- Parameters:
- filenamestr
Path of the .csv or .txt file with A, B, M and N electrodes. Electrode index start at 1.
- cmd_idstr, optional
Unique command identifier.
- Returns:
- sequencenumpy.ndarray
Array of shape (number quadrupoles * 4).
- plot_last_fw(save_fig=False, filename=None)
Plots last full waveform measurement
- Parameters:
- save_fig: boolean, optional - default (False)
Whether to save the figure
- filename: str, optional
Path to save plot. By default figures/test.png
- quit(cmd_id=None)
Quits OhmPi.
- Parameters:
- cmd_idstr, optional
Unique command identifier.
- remove_data(cmd_id=None)
Remove all data in the ´export_path´ folder on the raspberrypi.
- Parameters:
- cmd_idstr, optional
Unique command identifier.
- repeat_sequence(**kwargs)
Identical to run_multiple_sequences().
- reset_mux(cmd_id=None)
Switches off all multiplexer relays.
- Parameters:
- cmd_idstr, optional
Unique command identifier.
- restart(cmd_id=None)
Restarts the Raspberry Pi.
- Parameters:
- cmd_idstr, optional
Unique command identifier.
- rs_check(vab=5, cmd_id=None, couple=None, tx_volt=None)
Checks contact resistances. Strategy: we just open A and B, measure the current and using vAB set or assumed (12V assumed for battery), we compute Rab.
- Parameters:
- vabfloat, optional
Voltage of the injection.
- couplearray, for selecting a couple of electrode for checking resistance
- cmd_idstr, optional
Unique command identifier.
- tx_voltfloat, optional DEPRECATED
Save as vab.
- run_inversion(survey_names=None, elec_spacing=1, **kwargs)
Run a simple 2D inversion using ResIPy (https://gitlab.com/hkex/resipy).
- Parameters:
- survey_nameslist of string, optional
Filenames of the survey to be inverted (including extension).
- elec_spacingfloat (optional)
Electrode spacing in meters. We assume same electrode spacing everywhere. Default is 1 m.
- kwargsoptional
Additional keyword arguments passed to resipy.Project.invert(). For instance reg_mode == 0 for batch inversion, reg_mode == 2 for time-lapse inversion. See ResIPy document for more information on options available (https://hkex.gitlab.io/resipy/).
- Returns:
- xzvlist of dict
Each dictionnary with key ‘x’ and ‘z’ for the centroid of the elements and ‘v’ for the values in resistivity of the elements.
- run_measurement(quad=None, nb_stack=None, injection_duration=None, duty_cycle=None, strategy=None, vab_init=None, vab_min=None, vab_req=None, vab_max=None, iab_min=None, iab_req=None, min_agg=None, iab_max=None, vmn_min=None, vmn_req=None, vmn_max=None, pab_min=None, pab_req=None, pab_max=None, cmd_id=None, **kwargs)
Measures on a quadrupole and returns a dictionary with the transfer resistance.
- Parameters:
- quaditerable (list of int)
Quadrupole to measure, just for labelling. Only switch_mux_on/off really create the route to the electrodes.
- nb_stackint, optional
Number of stacks. A stack is considered two pulses (one positive, one negative). If 0, we will look for the best voltage.
- injection_durationint, optional
Injection time in seconds.
- duty_cyclefloat, optional
Duty cycle (default=0.5) of injection square wave.
- strategystr, optional, default: safe
Define injection strategy (if power is adjustable, otherwise safe vab, generally 12V battery is used). Either: - vmax : compute Vab to reach a maximum Vmn_max and Iab without exceeding vab_max - vmin : compute Vab to reach at least Vmn_min - safe : apply given Vab but checks if expected readings not out-of-range - fixed: apply given Vab with no out-of-range checks for optimising duration at the risk of out-of-range readings Safety check (i.e. short voltage pulses) performed prior to injection to ensure injection within bounds defined in vab_max, iab_max, vmn_max or vmn_min. This can adapt Vab. To bypass safety check before injection, vab should be set equal to vab_max (not recommended)
- vab_initfloat, optional
Initial injection voltage [V] Default value set by settings or system specs
- vab_minfloat, optional
Minimum injection voltage [V] Default value set by config or boards specs
- vab_reqfloat, optional
Requested injection voltage [V] Default value set by config or boards specs
- vab_maxfloat, optional
Maximum injection voltage [V] Default value set by config or boards specs
- iab_minfloat, optional
Minimum current [mA] Default value set by config or boards specs
- iab_reqfloat, optional
Requested iab [mA] Default value set by config or boards specs
- iab_maxfloat, optional
Maximum iab allowed [mA]. Default value set by config or boards specs
- pab_minfloat, optional
Minimum power [W]. Default value set by config or boards specs
- pab_reqfloat, optional
Requested power [W]. Default value set by config or boards specs
- pab_maxfloat, optional
Maximum power allowed [W]. Default value set by config or boards specs
- vmn_min: float, optional
Minimum Vmn [V] (used in strategy vmin). Default value set by config or boards specs
- vmn_req: float, optional
Requested Vmn [V] (used in strategy vmin). Default value set by config or boards specs
- vmn_max: float, optional
Maximum Vmn [V] (used in strategy vmin). Default value set by config or boards specs
- min_aggbool, optional, default: False
when set to True, requested values are aggregated with the ‘or’ operator, when False with the ‘and’ operator
- cmd_idstr, optional
Unique command identifier.
- run_multiple_sequences(sequence_delay=None, nb_meas=None, fw_in_zip=None, cmd_id=None, **kwargs)
- Runs multiple sequences in a separate thread for monitoring mode.
Can be stopped by ‘OhmPi.interrupt()’. Additional arguments are passed to run_measurement().
- Parameters:
- sequence_delayint, optional
Number of seconds at which the sequence must be started from each others.
- nb_measint, optional
Number of time the sequence must be repeated.
- fw_in_zipbool, optional
Whether to save the full-waveform data in a separate .csv in long format to be zipped to spare space. If None, default is read from default.json.
- cmd_idstr, optional
Unique command identifier.
- kwargsdict, optional
See help(OhmPi.run_measurement) for more info.
- run_sequence(fw_in_zip=None, cmd_id=None, save_strategy_fw=False, export_path=None, **kwargs)
- Runs sequence synchronously (=blocking on main thread).
Additional arguments (kwargs) are passed to run_measurement().
- Parameters:
- fw_in_zipbool, optional
Whether to save the full-waveform data in a separate .csv in long format to be zipped to spare space. If None, default is read from default.json.
- save_strategy_fwbool, optional
Whether to save the strategy used.
- export_pathstr, optional
Path where to save the results. Default taken from settings.json.
- cmd_idstr, optional
Unique command identifier.
- run_sequence_async(cmd_id=None, **kwargs)
- Runs the sequence in a separate thread. Can be stopped by ‘OhmPi.interrupt()’.
Additional arguments are passed to run_sequence().
- Parameters:
- cmd_idstr, optional
Unique command identifier.
- property sequence
Gets sequence
- set_sequence(sequence=None, cmd_id=None)
Sets the sequence to acquire.
- Parameters:
- sequencelist of list or array_like
Sequence of quadrupoles (list of list or array_like).
- cmd_id: str, optional
Unique command identifier.
- set_time(date, cmd_id=None)
Set date of the RPI remotely.
- Parameters:
- datestr
ISO datetime string such as 2024-07-23T20:00:01.345Z.
- cmd_idstr, optional
Unique command identifier.
- shutdown(cmd_id=None)
Shutdown the Raspberry Pi.
- Parameters:
- cmd_idstr, optional
Unique command identifier
- switch_mux_off(quadrupole, cmd_id=None)
Switches off multiplexer relays for given quadrupole.
- Parameters:
- quadrupolelist of 4 int
List of 4 integers representing the electrode numbers.
- cmd_idstr, optional
Unique command identifier.
- switch_mux_on(quadrupole, bypass_check=False, cmd_id=None)
Switches on multiplexer relays for given quadrupole.
- Parameters:
- quadrupolelist of 4 int
List of 4 integers representing the electrode numbers.
- bypass_check: bool, optional
Bypasses checks for A==M or A==N or B==M or B==N (i.e. used for rs-check).
- cmd_idstr, optional
Unique command identifier.
- test(test_names=[], remote=False, filename=None)
Run test on the ohmpi system.
- Parameters:
- test_nameslist, optional
Name of test to run. By default, all available test are run.
- remotebool, optional
If set to True, no input prompt will be presented to the user. It is assumed that the system can run this test remotely safely.
- filenamestr, optional
- Filepath with extension .json included. If specified, the results
of the tests will be saved there.
- test_mux(activation_time=0.2, mux_id=None, cmd_id=None)
Interactive method to test the multiplexer boards.
- Parameters:
- activation_timefloat, optional
Time in seconds during which the relays are activated.
- mux_idstr, optional
ID of the mux_board to test.
- cmd_idstr, optional
Unique command identifier.
- update_settings(settings: str, cmd_id=None)
Updates acquisition settings from a json file or dictionary. Parameters can be: - nb_electrodes (number of electrode used, if 4, no MUX needed) - injection_duration (in seconds) - nb_meas (total number of times the sequence will be run) - sequence_delay (delay in second between each sequence run) - nb_stack (number of stack for each quadrupole measurement) - strategy (injection strategy: safe, vmax, vmin) - duty_cycle (injection duty cycle comprised between 0.5 - 1) - export_path (path where to export the data, timestamp will be added to filename)
- Parameters:
- settingsstr, dict
Path to the .json settings file or dictionary of settings.
- cmd_idstr, optional
Unique command identifier.
- class ohmpi.hardware_system.OhmPiHardware(**kwargs)
OhmPiHardware class. A class to operate the system of assembled components as defined in the ohmpi/config.py file
- Attributes:
- pulses
- pwr_state
Methods
compute_vab
([vab_init, vab_min, vab_req, ...])Estimates best Vab voltage based on different strategies.
Switches off all multiplexer relays.
switch_mux
(electrodes[, roles, state])Switches on multiplexer relays for given quadrupole.
test_mux
([channel, activation_time])Interactive method to test the multiplexer.
vab_square_wave
(vab, cycle_duration[, ...])Performs a Vab injection following a square wave and records full waveform data.
calibrate_rx_bias
discharge_pwr
last_dev
last_iab
last_iab_dev
last_resistance
last_sp
last_vmn
last_vmn_dev
select_samples
- compute_vab(vab_init=5.0, vab_min=None, vab_req=None, vab_max=None, iab_min=None, iab_req=None, iab_max=None, vmn_min=None, vmn_req=None, vmn_max=None, pab_min=None, pab_req=None, pab_max=None, min_agg=False, polarities=(1, -1), pulse_duration=0.1, delay=0.0, diff_vab_lim=2.5, n_steps=4, n_sigma=2.0, filename=None, quad_id=0)
Estimates best Vab voltage based on different strategies. In “vmax” and “vmin” strategies, we iteratively increase/decrease the vab while checking vmn < vmn_max, vmn > vmn_min and iab < iab_max. We do a maximum of n_steps and when the difference between the two steps is below diff_vab_lim or we reached the maximum number of steps, we return the vab found.
- Parameters:
- pulse_durationfloat, optional
Time in seconds for the pulse used to compute optimal Vab.
- vab_initfloat, optional
Initial voltage to search for best vab.
- vab_maxfloat, optional
Maximum injection voltage to apply to tx (used by all strategies).
- vmn_maxfloat, optional
Maximum voltage target for rx (used by vmax strategy).
- vmn_minfloat, optional
Minimum voltage target for rx (used by vmin strategy).
- polaritieslist of int, optional
Polarity of the AB injection used to compute optimal Vab. Default is one positive, then one negative.
- p_maxfloat, optional
Maximum power that the device can support/sustain.
- diff_vab_limfloat, optional
Minimal change in vab between steps for continuing the search for optimal vab. If change between two steps is below the diff_vab_lim, we have found the optimal vab.
- n_stepsint, optional
Number of steps to try to find optimal vab. Each step last at least injection_duration*len(polarities) seconds.
- Returns:
- vabfloat
Proposed Vab according to the given strategy.
- reset_mux()
Switches off all multiplexer relays.
- switch_mux(electrodes, roles=None, state='off', **kwargs)
Switches on multiplexer relays for given quadrupole.
- Parameters:
- electrodeslist
List of integers representing the electrode ids.
- roleslist, optional
List of roles of electrodes, optional
- statestr, optional
Either ‘on’ or ‘off’.
- test_mux(channel=None, activation_time=1.0)
Interactive method to test the multiplexer.
- Parameters:
- channeltuple, optional
(electrode_nr, role) to test.
- activation_timefloat, optional
Time in seconds during which the relays are activated.
- vab_square_wave(vab, cycle_duration, sampling_rate=None, cycles=3, polarity=1, duty_cycle=1.0, append=False)
Performs a Vab injection following a square wave and records full waveform data. Calls in function Vab_pulses.
- Parameters:
- vab: float
Injection voltage [V]
- cycle_duration: float
Duration of one cycle within the square wave (in seconds)
- sampling_rate: float, None Default None
Sampling rate for Rx readings
- cycles: integer, Default: 3
Number of cycles
- polarity: 1, 0 , -1
Starting polarity
- duty_cycle: float (0 to 1)
Duty cycle of injection wave
- append: bool, optional
Default: False